Embed SDK Java
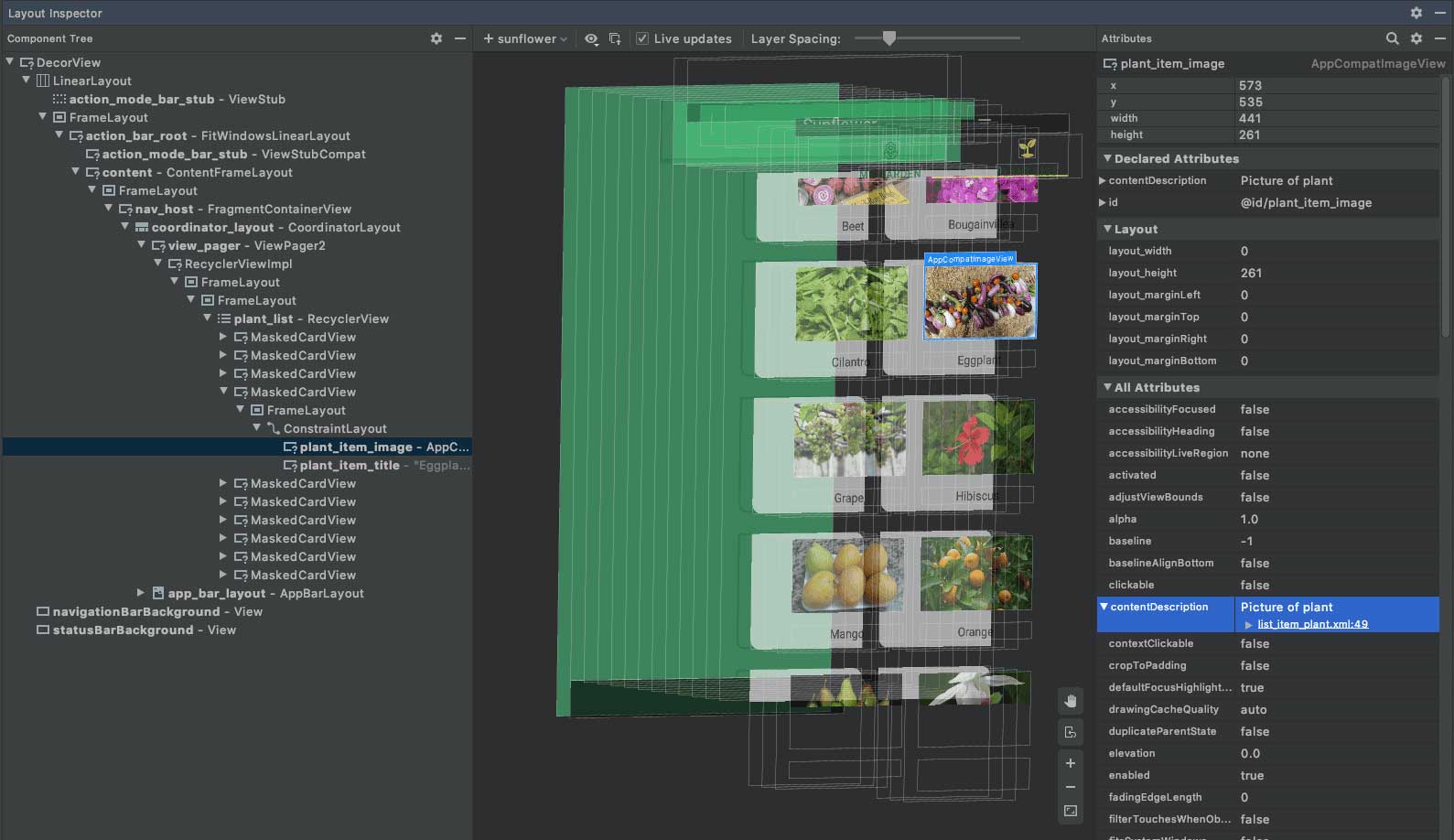
Download the XRMOD AAR
The first step to enhancing your application is to get our SDK! We have uploaded XRMOD Framework to github. You can click here to go to the github page and download our XRMOD AAR. If you think XRMOD is good please give us a star. Thank you!
Import XRMOD AAR
Select your project's app
folder, via the context menu Reveal in Finder (Mac)
or Show in Explorer (Windows)
. Place all downloaded AAR files in app/libs/
.
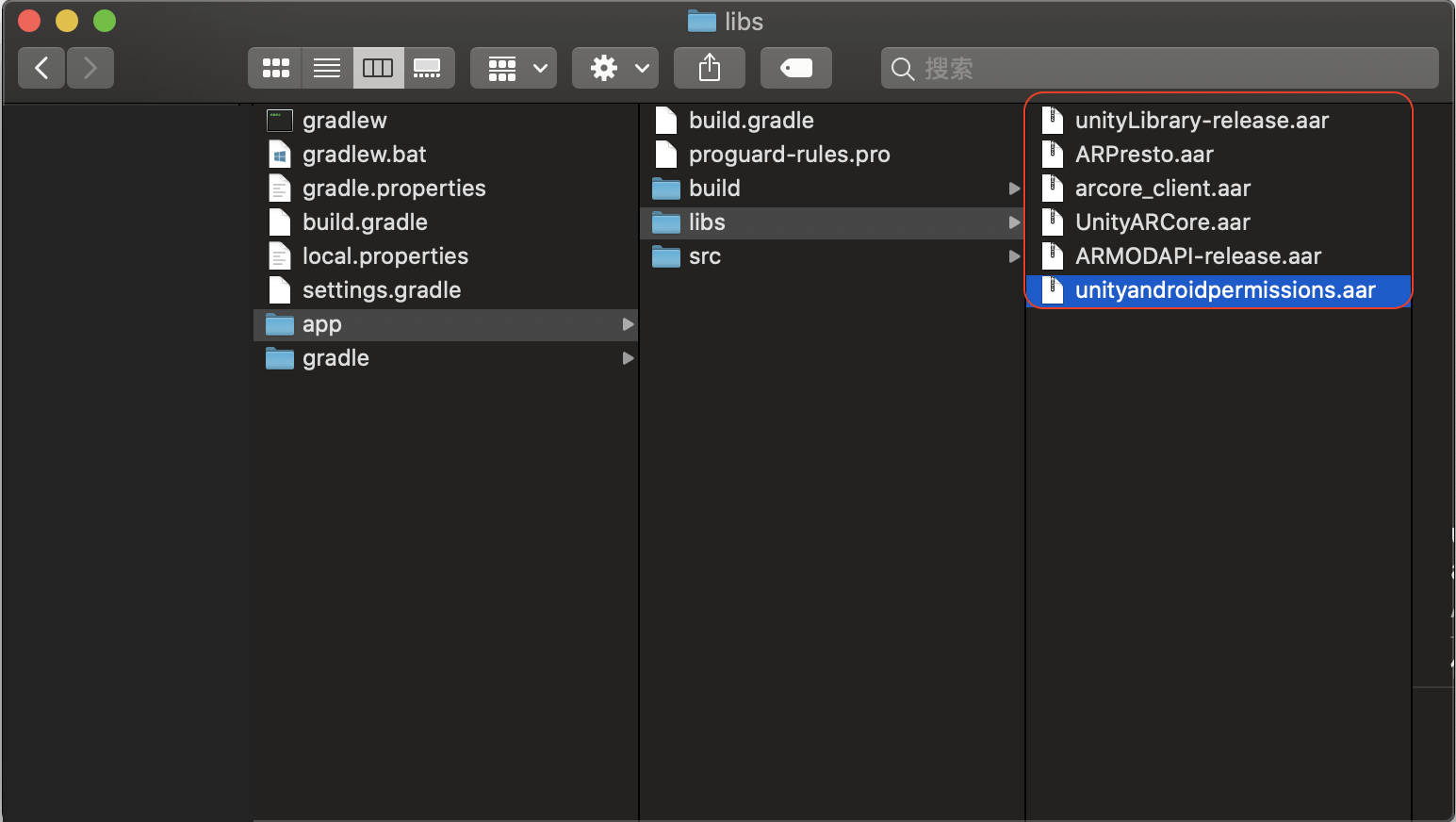
Image | Name | Format | Brief |
![]() | |||
unityLibrary-release | AAR | Main library | |
XRMODAPI-release | AAR | All API | |
UnityARCore | AAR | ARCore | |
arcore_client | AAR | ARCore | |
ARPresto | AAR | ARCore | |
unityandroidpermissions | AAR | ARCore |
Embed Coding
NDK Build Configure
Back to Android Studio Editor. And Edit build.gradle(Module:YOUR_PROJECT_NAME.app)
. Append some configre's code.
Adding NDK to android
-> defaultConfig
block.
ndk {
abiFilters 'armeabi-v7a', 'x86', 'arm64-v8a'
}
android {
...
defaultConfig {
...
//New config
ndk {
abiFilters 'armeabi-v7a', 'x86', 'arm64-v8a'
}
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
...
}
Please set up according to the architecture you need
Dependencies setup
Adding dependencies. You can also use implementation(name:'AAR_FILE_NAME',ext:'aar')
to import new dependencies.
implementation fileTree(dir: 'libs', include: ['*.jar','*.aar'])
dependencies {
//New dependencies
implementation fileTree(dir: 'libs', include: ['*.jar','*.aar'])
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'com.google.android.material:material:1.2.1'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
implementation 'androidx.navigation:navigation-fragment:2.3.1'
implementation 'androidx.navigation:navigation-ui:2.3.1'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
Sync your gradle!
> Task :prepareKotlinBuildScriptModel UP-TO-DATE
BUILD SUCCESSFUL in 762ms
Implement Callback
You must implement all abstract methods. These are callbacks of the XRMOD SDK.
Launch AR
Create a new Activity
call it ARView
. Then extends AbstractXRMODActivity
and implementing abstract method onCreateUI
Go to , adding the ARView
activity in application
block.
<activity android:name=".ARView" android:screenOrientation="fullSensor" android:configChanges="mcc|mnc|locale|touchscreen|keyboard|keyboardHidden|navigation|orientation|screenLayout|uiMode|screenSize|smallestScreenSize|fontScale|layoutDirection|density" android:hardwareAccelerated="false" android:process=":Unity"
android:label="@string/app_name">
</activity>
Add some code to onCreateUI function.
//Init sdk
initXRMOD("JSON Config",MainActivity.class);
//Add UI from layout file
LayoutInflater tmp_Inflater = getLayoutInflater();
View tmp_ARView = tmp_Inflater.inflate(R.layout.arview_main,null);
getXRMODFrameLayout().addView(tmp_ARView);
Add new function to stop the ARView.
public void CloseARView(View _v){
unloadAndHideXRMOD();
}
Back to MainActivity.java, adding a new function to call the sdk.
public void ARLauncher(View _v){}
Using the Intent to start a new activity.
Intent intent = new Intent(this, ARView.class);
intent.setFlags(Intent.FLAG_ACTIVITY_REORDER_TO_FRONT);
startActivityForResult(intent, 1);
Summary
More infomation of Native API you should read Android Native Calls Protocol API. We uploaded the sample project of this Native Android (Java) project to github. At now you can download our source project from github.