How To Load Assets
The XRMOD engine allows creators to load custom assets via CSharp scripts or visual scripts.
But, how should we do that?
You must install Unity Engine and Dev-environment.
At the first, we need to create a XRMOD project(How to create).
How to find XRMOD project after created?
click here to know more
Collection Assets
Creative can import their own art assets or use Unity builtin assets(Primitive Objects).
click here to know about Unity how to import assets.
Follows Steps:
- Import your art assets into
Your Project/Artwork
folder - Create a new folder and name it to
Prefabs
. This folder will be used to store the project's prefabs - Drag-and-drop art asset into Unity Scene or Hierarchy
- Processing your art assets, e.g. Rendering of models, mapping, scale, etc
- Drag-and-drop your art asset from Unity Scene or Hierarch to
Your Project/Artwork/Prefabs
folder, it will become a prefab - Drag-and-drop your prefab of art work to PackageToolsEditor->Contents
Editing Script
Go to PROJECT_PATH
->PROJECT_NAME
->Scripts
->Runtime
and open PROJECTNAMEMainEntry.cs
file(By double-clicking).
Then write some code on OnLoad
meethod.
ARMODAPI.LoadAssetAsync<GameObject>("VirtualObject"); The object name(VirtualObject
) must be the same as in PackageToolsEditor
->Contents
assets name.
using System;
using UnityEngine;
using System.Collections;
using com.Phantoms.ARMODAPI.Runtime;
using com.Phantoms.ActionNotification.Runtime;
using Object = UnityEngine.Object;
namespace HowToCreateProject.Runtime
{
public class HowToCreateProjectMainEntry
{
//XRMOD API
internal static API ARMODAPI = new API(nameof(HowToCreateProject));
public async void OnLoad()
{
var tmp_ARVirtualObjectPrefab = await ARMODAPI.LoadAssetAsync<GameObject>("VirtualObject");
Object.Instantiate(tmp_ARVirtualObjectPrefab);
}
}
}
We use LoadAssetAsync
to load our virtual objects from the XR-Experience package by asset name and type. Because the LoadAssetAsync
method loads the asset from disk to memory, it does not instantiate the asset, so we need to call Instantiate
method to instantiate it.
Programmable Block
- Go to PackageToolsEditor
- Switch to Properties section
- Move your mouse point to free area
- Right click your mouse button
- Select
Programmable
Build XR-Experience
- Go to PackageToolsEditor
- Switch to Build section
- Select the platform you need to build.
- Click the Build AR-Experience button to start the build
Build Platform and Platform Group The two selected platforms must be the same.
Testing
Now we have done all the work, but we don't know if the content loads properly yet, so we need to test further to verify our results.
How to testing?
XRMOD Engine currently provides processes such as loading tests within the Unity Editor, so we can verify all logic from within the Unity Editor. Before we can start testing we need to install the XRMOD Engine Simulator plugin via the Unity Package Manager.
Click here to learn how to install the XRMOD engine toolchain.
In here, we need to install XRMOD Engine Simulator plugin.
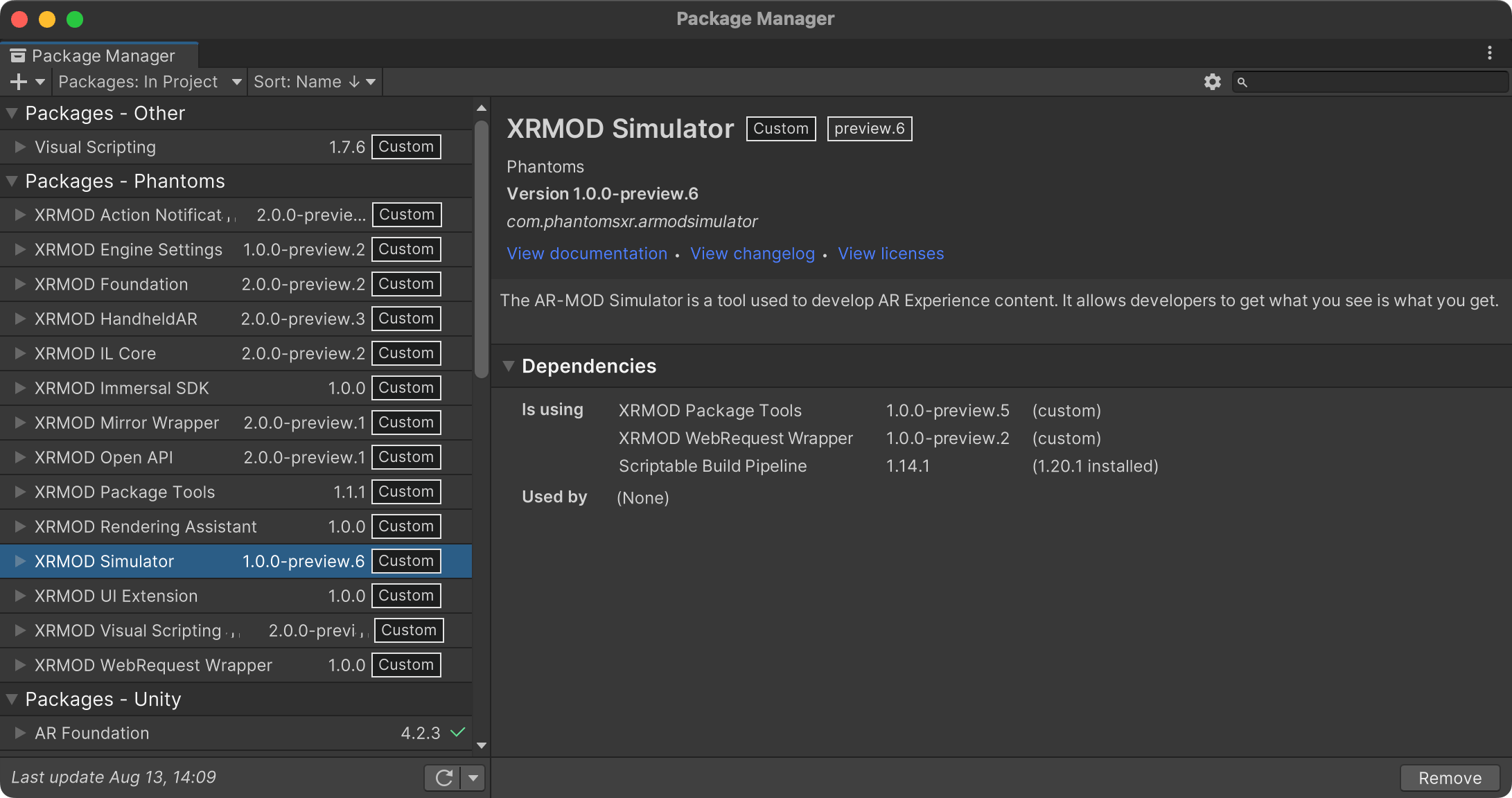
When you finish the installation you can see an XR button in the top left corner of the Unity editor, which is mainly used to start the XRMOD Engine emulator.

Launch Simulator
- Click left-top XR Play button to launch XRMOD Engine simulator
- Switch to Game view
- Typing your project name into simulator
- Click Fetch Project to loading our project
Summary
In this section we learned how to load an assets in XRMOD Engine. During the loading process we need to pay attention to the following:
- Loading asset via
ARMODAPI.LoadAssetAsync
methods ARMODAPI.LoadAssetAsync
needs to pass in the type to be loaded and the name of the item to be loadedARMODAPI.LoadAssetAsync
is an asynchronous method that needs to be marked as async in the function and addawait
before theARMODAPI.LoadAssetAsync
method- The name of the object loaded in the API must be the same as the name of the assets in
PackageToolsEditor
->Contents